Boto3 is a comprehensive Python library for interacting with AWS services. It provides high-level APIs for a wide range of AWS services, including Amazon S3, Amazon EC2, Amazon DynamoDB, and many more. Boto3 is easy to use and can be used to automate tasks, build custom tools, and develop applications that interact with AWS.
Boto3 uses the security credentials to connect with the AWS services. While connecting the AWS, the error NoCredentialsError: Unable to locate credentials
occurs when it cannot find the credentials needed to connect to AWS.
This can happen for a number of reasons, such as:
- The credentials are not set in the environment
- The credentials are not stored in the default location
- The credentials are invalid
Quick Solution: Configure the credentials using the aws configure
command.
aws configure
Table of Contents
Causes Of the NoCredentialsError: Unable to Locate Credentials
The following are some of the possible causes of the error NoCredentialsError: Unable to locate credentials
:
- The AWS_ACCESS_KEY_ID and AWS_SECRET_ACCESS_KEY environment variables are not set
- The
~/.aws/credentials
file does not contain the required credentials - The credentials in the
~/.aws/credentials
file are invalid or not accessible
Prerequisite to Fix the NoCredentialsError: Unable to Locate Credentials
- Security credentials AWS Access Key ID and the Secret Access Key.
The Security credentials can be created by clicking your Profile name at the top right corner, Clicking the Security credentials menu, and clicking the Create access key option available under the Access keys section.
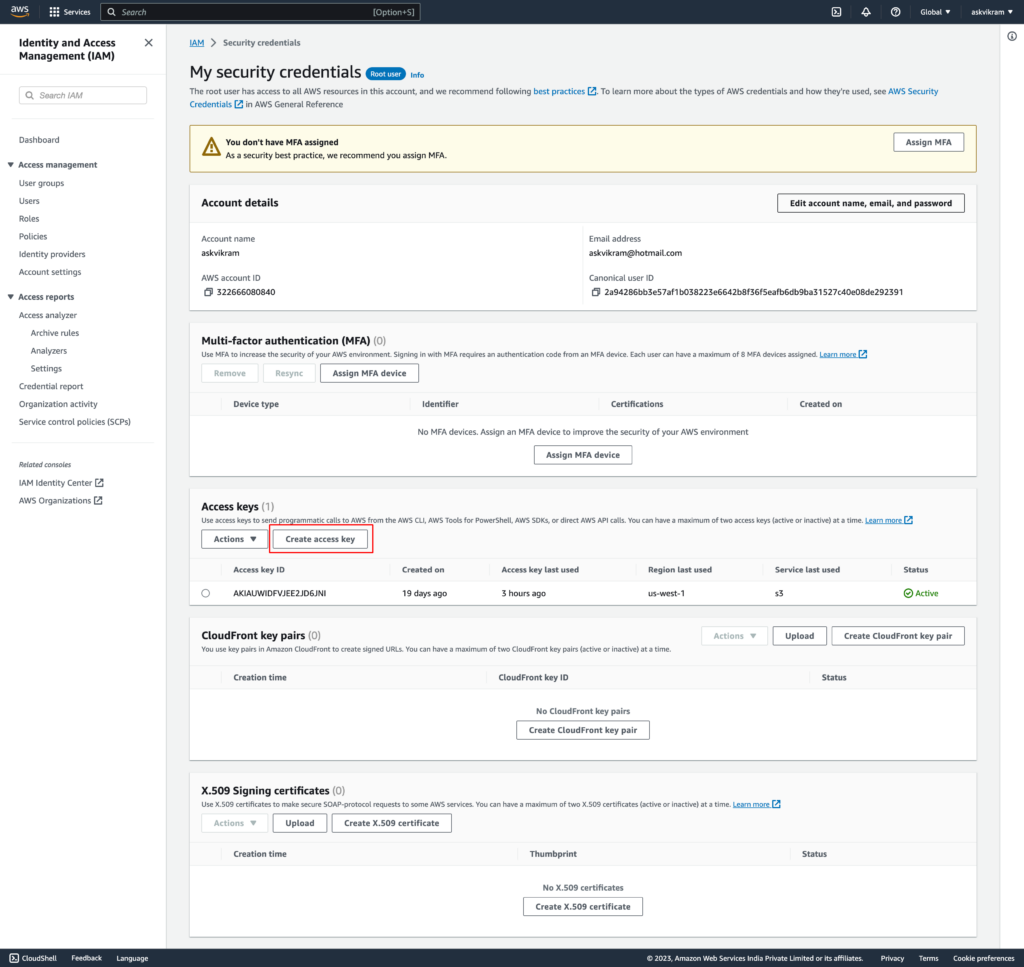
This is how you can create the security credentials.
How to fix the NoCredentialsError: Unable to Locate Credentials
To fix the error NoCredentialsError: Unable to locate credentials
, you must provide Boto3 with the credentials it needs to connect to AWS. Different ways are there to do this.
Option 1: Configuring the Security Credentials Using AWS Configure
You can configure the Security credentials using the configure
command as shown below.
aws configure
When you press Enter
, you’ll be prompted to enter the AWS access key and secret access keys individually. Once done, the details will be saved on the ~/.aws/credentials
file.
Option 2: Configuring the Security Credentials Using Environment Variables
To configure the environment variables in Linux or Mac,
- Open the terminal window and enter the following comments one by one.
export AWS_ACCESS_KEY_ID=<your-access-key>
export AWS_SECRET_ACCESS_KEY=<your-secret-key>
To make these changes permanent, add these export commands to your shell’s configuration file (e.g., ~/.bashrc
for Bash or ~/.zshrc
for Zsh). This ensures the environment variables are set every time you open a new terminal session.
To configure the environment variables in Windows,
- Create two environment variables as user variables with the names
AWS_ACCESS_KEY_ID
andAWS_SECRET_ACCESS_KEY
and store the values appropriately.
Now, the environment variables are set on your system, and it allows AWS CLI or the SDKs to interact with your AWS account services securely.
Specifying the Security Credentials in your Program
As an alternative solution, you can specify the Security credentials in your program while creating a Boto3 session.
It is not a best practice from a security standpoint to hardcode the security credentials in your program.
Code
The following code demonstrates how to specify credentials while creating a Boto3 session. You can use the session object to create a client
or a resource
object.
import boto3
session = boto3.Session(
aws_access_key_id='Your Access Key ID',
aws_secret_access_key='Your Secret Access Key'
)
s3_client = session.client('s3')
## Additional Code to access the AWS resource using the S3 client.
Specifying Security Credentials While Using Docker Service
To configure the credentials as environment variables before initializing the Docker client, follow these steps:
- Open your Docker environment
- Set the environment variables using the
ENV
command in your Dockerfile to set the aws_access_key_id and aws_secret_access_key environment variables as shown below.
ENV aws_access_key_id=<your-access-key>
ENV aws_secret_access_key=<your-secret-key>
- Save the Dockerfile and build your Docker image using the
docker build
command. This will ensure that the specified AWS credentials are included in the image. - When you run Docker containers from this image, they will have access to the AWS credentials as environment variables. You can then use these credentials within your containers to interact with AWS services securely.
By setting AWS security credentials as environment variables within your Docker image, you can ensure that your Dockerized applications have the necessary access to AWS resources while maintaining security and isolation within your containerized environment.
Additional Tips for Troubleshooting the Error
- Make sure that you are using the correct region
- Make sure that you are using the correct AWS service
- Try using a different IAM user
Conclusion
In this tutorial, you learned how to fix the Boto3 NoCredentialsError: Unable to Locate Credentials
error. You learned about the causes of the errors and how to fix them.
Here are some additional things to keep in mind:
- If you are using a shared computer, ensure you do not share your security credentials with others.
- If you are storing your security credentials in a file, make sure that the file is encrypted and that only you have access to it.
- If you are using the Docker service, ensure you only allow trusted users to access your Docker images.
- AWS access keys and secret keys may need to be updated regularly, especially if credentials are shared or compromised to keep your account secure.
By following these tips, you can help to keep your security credentials safe and secure.